Create Node Tools#
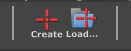
Creating various nodes and objects with mmSolver uses the tools below.
Create Marker#
Create a default Marker node under the active viewport’s Camera.
Usage:
Click in a Maya 3D viewport, to activate the Camera.
Run the Create Marker tool, using the shelf, menu or marking menu.
A Marker will be created in the center of the viewport.
Note
Default Maya cameras (such as persp
, top
, front
, etc)
are not supported to create Markers.
Note
To create Markers, with 2D data from external 3D software use the Load Markers tool.
Run this Python command:
import mmSolver.tools.createmarker.tool as tool
tool.main()
Create Bundle#
Create a default Bundle node.
If Markers are selected, the Bundle will attempt to attach to it, while adhering to the rule; a bundle can only have one marker representation for each camera.
Run this Python command:
import mmSolver.tools.createbundle.tool as tool
tool.main()
Create Line#
Create a 2D Line node, made up of Markers and Bundles. The Line defines a straight line between all Markers on the Line.
Adjust the Marker positions to move the Line.
Note
To display a straight line select the mmLineShape
shape
node and enable Draw Middle.
Run this Python command:
import mmSolver.tools.createline.tool as tool
tool.main()
Create Camera#
Create a default Camera.
Run this Python command:
import mmSolver.tools.createcamera.tool as tool
tool.main()
Create Lens#
Create a Lens (distortion) node.
The Lens node is used to distort all Markers attached to the Camera similar to how imperfections in physical lenses distorts light (mostly at the edges) of an image.
Usage:
Activate 3D viewport or select camera node(s).
Run the Create Lens tool, using the shelf or menu.
Adjust lens node attributes as needed, or load a lens with the Load Markers in Maya.
Run this Python command:
import mmSolver.tools.createlens.tool as tool
tool.main()
Create ImagePlane#
Create a MM ImagePlane node, with the chosen image file (sequence) used to display a flat plane with an image texture in the Maya 3D scene.
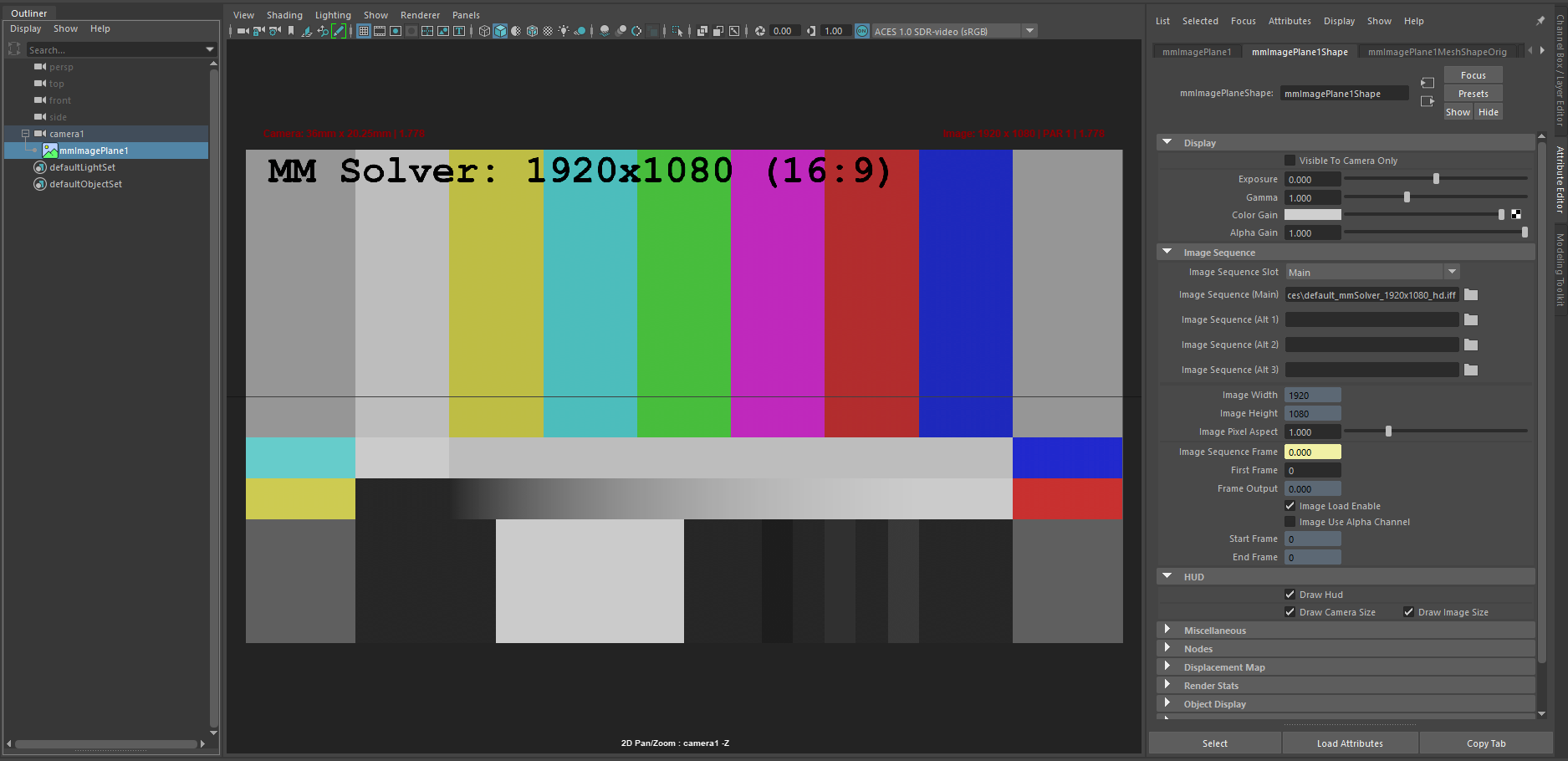
Usage:
Activate 3D viewport or select camera node(s).
Run the Create ImagePlane tool, using the shelf or menu.
Browse to image file.
If the image is named as an image sequence (such as
file.#.ext
), it will be detected and the full image sequence will be loaded.
To create an image plane, you can run this Python command:
import mmSolver.tools.createimageplane.tool as tool
tool.main()
MM ImagePlane#
The MM ImagePlane node is an improved Image Plane, designed for MatchMove tasks, and can be created with the Create ImagePlane tool.
Key features:
Multiple Image Slots; Switch seamlessly between 4 different image sequences loaded onto the MM ImagePlane.
Memory resource control; The Image Cache is used to limit and detail memory usage for the MM ImagePlane allowing greater control than the native Maya ImagePlane.
Real-Time Lens Distortion; Lenses added to the camera (with Create Lens tool) will distort the MM ImagePlane in real-time as attributes update.
Frame Range controls and details; Override the first frame of an image sequence to any other frame, and see the output frame number easily for debugging.
Enhanced Display controls; Adjust the exposure, gamma, saturation and soft-clip of the input image data, and display individual colour channels.
Display Attributes#
Display Attributes control how the MM ImagePlane looks in the viewport; use these attributes to make the image easier to see.
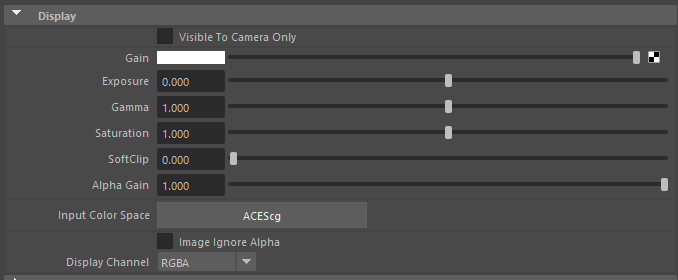
Name |
Description |
---|---|
Visible To Camera Only |
When enabled, this imagePlane node is not visible when viewed
by any other camera (including |
Gain |
The color of multiplier of the displayed image. |
Exposure |
Relative exposure brightness (measured in Stops) applied to the image. Negative numbers darken the image, positive values increase the brightness. |
Gamma |
Brighten or darken the mid-tones of the image, without affecting the shadows or highlights as much. Do not use this slider to approximate a Color Space of 2.2 - use the Input Color Space attribute. |
Saturation |
Adjust the color saturation of the image; 0.0 makes the image black and white, 1.0+ numbers make the image colors very vibrant. |
SoftClip |
Reduce the image highlights to flatten the bright areas of an image. |
Alpha Gain |
Alpha channel multiplier. Controls the opacity/transparency of the image. |
Input Color Space |
The color space saved into the image. Left-click to choose color space from menu. |
Image Ignore Alpha |
If the image contains an alpha channel, you can ignore it here, and treat the image as fully opaque. |
Display Channel |
Which channel the image would you like to display? |
Image Sequence Attributes#
Image Sequence attributes define the image that is loaded and the frame number used for image look-up.
MM ImagePlane is designed to contain multiple image sequence Slots, so that users can easily swap between different images. For example, you may have an EXR image sequence, a lower-resolution JPEG image sequence and a color adjusted image sequence to better see low-contrast image details - you can easily swap between all of these image sequences by changing the Image Sequence Slot attribute.
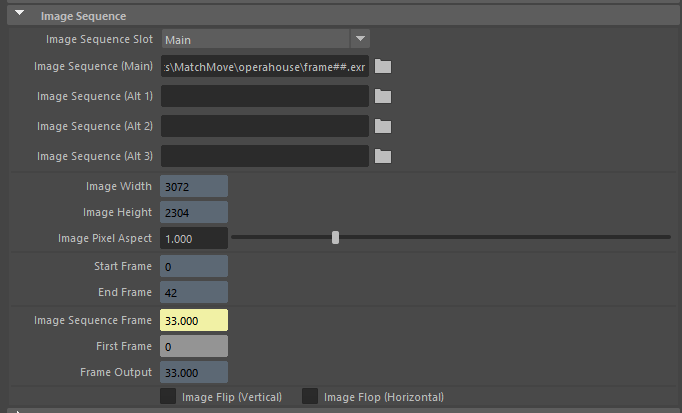
Name |
Description |
---|---|
Image Sequence Slot |
Change the Image Sequence Slot, between the 4 image sequences
available; |
Image Sequence (Main) |
The “Main” image sequence, and the default. |
Image Sequence (Alt 1) |
First alternate image sequence. |
Image Sequence (Alt 2) |
Second alternate image sequence. |
Image Sequence (Alt 3) |
Third alternate image sequence. |
Image Width |
Resolution width of loaded image. |
Image Height |
Resolution height of loaded image. |
Image Pixel Aspect |
Pixel-aspect ratio loaded image. This changes the way the
physical image plane size is calculated. For example, when
loading raw un-squeezed Anamorphic images, set this value to
|
Start Frame |
The start frame of the loaded image sequence. Will be |
End Frame |
The end frame of the loaded image sequence. Will be |
Image Sequence Frame |
The current input image sequence frame number. |
First Frame |
What frame is the first frame in the image sequence? MM ImagePlane Offsets the Start Frame of the image sequence so it is displayed when the Maya timeline is at First Frame. |
Frame Output |
The current output image sequence frame number. |
Image Flip (Vertical) |
Flips the image vertically; does not flip any asymmetric lens distortion values. |
Image Flop (Horizontal) |
Flops the image horizontally; does not flop any asymmetric lens distortion values. |
HUD (Heads-Up Display) Attributes#
HUD (Heads-Up Display) attributes control the way text is drawn in the viewport when the MM ImagePlane is viewed from it’s connected camera.
The HUD displays useful information intended to help understanding of the loaded image and help spot common issues.

Name |
Description |
---|---|
Draw Hud |
Enable or disable all HUD features. |
Draw Camera Size |
Enable or disable the camera size display. |
Draw Image Size |
Enable or disable the image size display. |
Image Cache Attributes#
Provides quick access to see details and control the Image Cache, and clear memory resources.
See Image Cache Preferences for details on how to control the MM Image Plane hardware resources used.
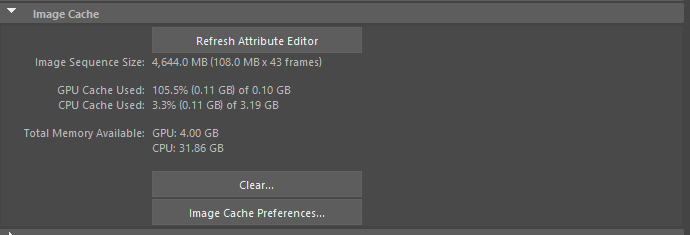
Name |
Description |
---|---|
Refresh Attribute Editor (button) |
Update the text fields in the attribute editor. |
Image Sequence Size |
Breaks down the amount of memory required for the full image sequence currently loaded; the size of a single frame multiplied by the image sequence frame count. |
GPU Cache Used |
Amount of GPU cache capacity is currently used. |
CPU Cache Used |
Amount of CPU cache capacity is currently used. |
Total Memory Available |
Amount of memory resources available on the computer. |
Clear… (button) |
Left-click to open a menu; clearing all images, clearing memory from image sequence slots on the current MM ImagePlane node. |
Image Cache Preferences… (button) |
Left-click to open the Image Cache Preferences. |
Miscellaneous Attributes#
Miscellaneous attributes do not fit into the other categories and are not commonly adjusted, but provided for rare use cases.

Name |
Description |
---|---|
Mesh Resolution |
Used to adjust the real-time lens distortion quality. You should adjust the resolution with more polygons when lens distortion is so extreme that the lens distortion is displayed is not accurate. Increasing the number also slows-down the real-time performance. |
Output Color Space |
This is the color space that Maya should work with internally. Left-click to choose color space from menu. |
Nodes Attributes#
These attributes can be used to quickly navigate to the connected nodes of this MM ImagePlane node.
The node connections cannot be adjusted using this UI. Only adjust these attributes if you know what you are doing, or want to experiment.

Name |
Description |
---|---|
Geometry Node |
Mostly used for debugging. The connected node used to extract mesh geometry for the MM ImagePlane to draw on the screen. This geometry node may be deformed by lens distortion. |
Camera Node |
The image plane is attached to this camera node. |
Extended Image Details Attributes#
Extended Image Details explain the internal and low-level details of the loaded images, and they are used to debug and for scripting tools.
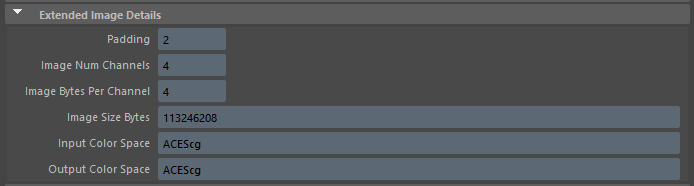
Name |
Description |
---|---|
Padding |
Image sequence file path frame number padding; For example
|
Image Num Channels |
The number of channels saved in the loaded image; 3 for RGB, 4 for RGBA. |
Image Bytes Per Channel |
The number of bytes that is used for each image channel. 8-bit is 1-byte; 32-bit is 4-bytes. |
Image Size Bytes |
The calculated number of bytes for a single image. This value is used to make calculations for the Image Cache Attributes attributes, and is mostly intended for debugging and scripting. |
Input Color Space |
The string value of the Input Color Space of the loaded image. Used for debugging. |
Output Color Space |
The string value of the Output Color Space of the loaded image. Used for debugging. |